Do you have a custom API that you use in your company internally and would like to connect to your favorite analytics tool, or integrate with any other tool using standards based connectivity like JDBC?
Do you have a data source which has a REST API and doesn’t have a JDBC driver, but you would like to have one for your analytics or integration purposes?
If so, you're at the right place. This tutorial will get you started in building your own JDBC driver using Progress DataDirect OpenAccess SDK. In this tutorial, I will build a JDBC driver for a free financial REST API provided by Alpha Vantage, which offers real time and historical stock market data.
Update: For a code-less solution, look at our new Autonomous REST Connector, which is designed to connect to any REST API easily. You can get started by following this tutorial.
- To test the driver, I would be using a free SQL query tool called SQL Workbench. Add the OpenAccess JDBC driver that you have installed earlier as shown below.
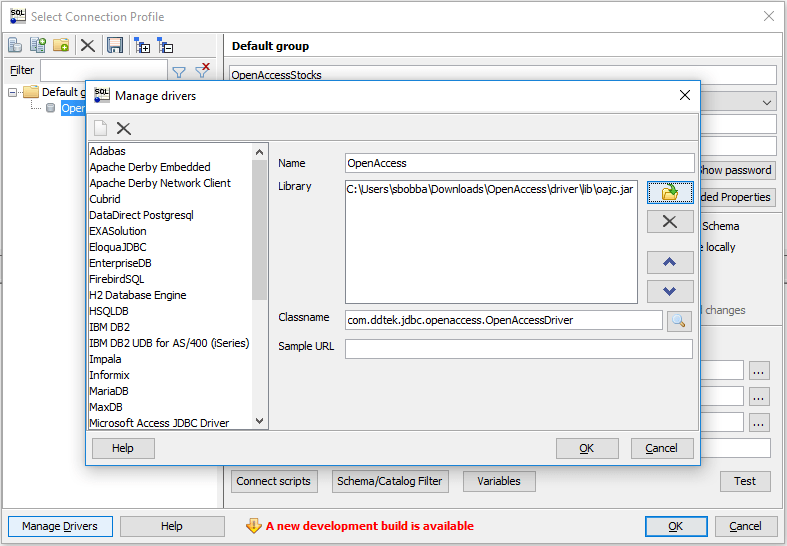
- Configure the OpenAccess driver as shown below and click on OK.
jdbc:openaccess://localhost:<port>;CustomProperties=(apikey=<key>)
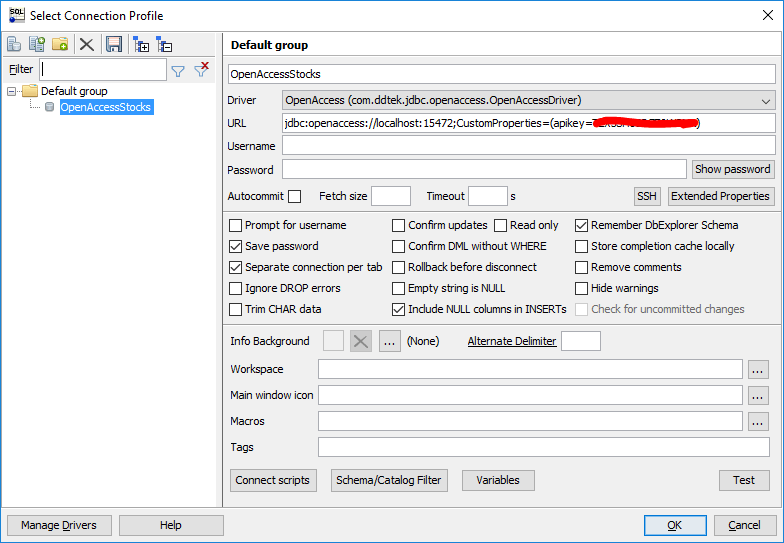
- You should now be able to run queries. If you have started debugging in IDE and placed breakpoints, you should be able to debug too simultaneously.
- Here are some sample queries you should be able to run, if you have used my code from GitHub.
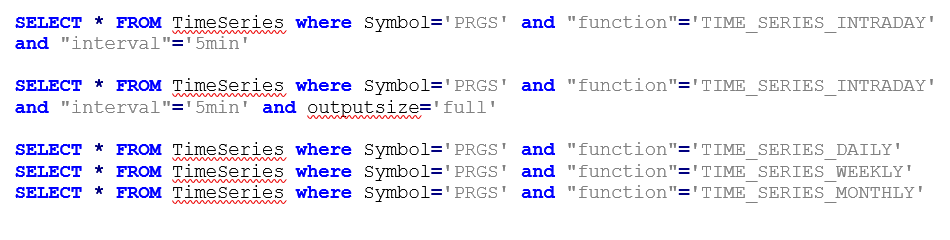
We hope this tutorial helped you to build your own custom ODBC driver using
Progress DataDirect OpenAccess SDK. If you have any questions/issues, feel free to reach out to us, we will be happy to help you during your evaluation.