Connecting to databases is one of the common things that developers want to do from any language. In this tutorial, we will walk you through how you can connect to SQL Server from Clojure using the clojure.java.jdbc
library. Note that this tutorial can be used with databases like Oracle, Postgres, DB2 or in fact for any source you have a JDBC driver. We will be showing you all CRUD operations in this tutorial for you to get started.
Download Leiningen script from
official websitechmod a+x ~/bin/lein
Execute lein, and it will download the self-install package
$ lein
DataDirect SQL Server JDBC driver.Install SQL Server JDBC driver by running the following command
java -jar PROGRESS_DATADIRECT_JDBC_SQLSERVER_ALL.jar
Follow through the prompts of Installer and install the driver in default path or custom path.
To add the JDBC driver to Clojure classpath, you need to add it to the Maven repository by running the following command.
mvn install:install-file
"-DgroupId=com.ddtek.jdbc"
"-DartifactId=sqlserver"
"-Dversion=1.0.0"
"-Dpackaging=jar"
"-Dfile=/path/Progress/DataDirect/Connect_for_JDBC_51/lib/sqlserver.jar"
Now, when you go to the maven repository, you should see the DataDirect SQL Server JDBC driver there as shown below.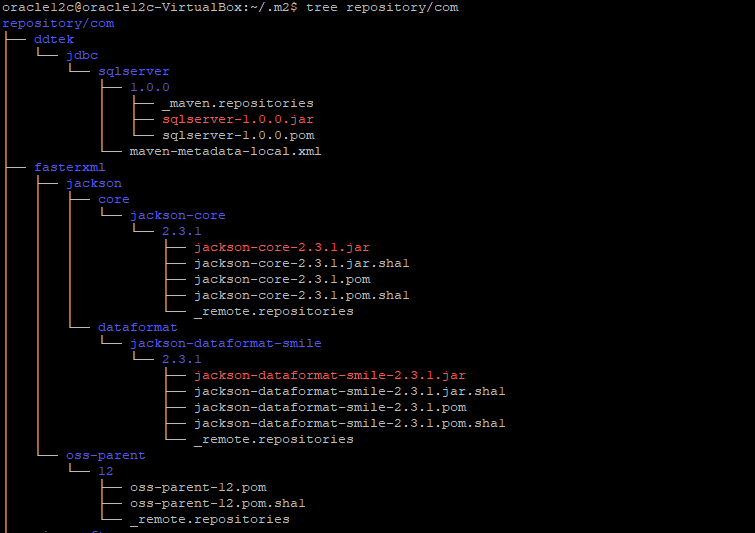
lein new app <app
-
name>
:dependencies [[org.clojure
/
clojure
"1.8.0"
]
[org.clojure
/
java.jdbc
"0.7.5"
]
[com.ddtek.jdbc
/
sqlserver
"1.0.0"
]]
:plugins [[lein
-
gorilla
"0.4.0"
]]
lein gorilla :ip
0.0
.
0.0
(ns sqlserver
-
connect
(:require [clojure.string :as str]
[clojure.java.jdbc :as j]))
(
def
db {:classname
"com.ddtek.jdbc.sqlserver.SQLServerDriver"
:subprotocol
"datadirect:sqlserver"
:subname
"//<host>:<port>;databaseName=<db>;user=<user>;password=<user>"
})
println db
(
def
pokemon
-
sql (j
/
create
-
table
-
ddl :pokemon [
[:pokedexid
"int"
"PRIMARY KEY"
]
[:pokemon_name
"VARCHAR(30)"
]
[:type1
"VARCHAR(15)"
]
[:type2
"VARCHAR(15)"
]]))
println pokemon
-
sql
(j
/
execute! db [pokemon
-
sql])
(j
/
insert
-
multi! db :pokemon [
{:pokedexid
1
:pokemon_name
"Bulbasaur"
:type1
"grass"
:type2
"poison"
}
{:pokedexid
2
:pokemon_name
"Ivysaur"
:type1
"grass"
:type2
"poison"
}
{:pokedexid
3
:pokemon_name
"Venusaur"
:type1
"grass"
:type2
"poison"
}
{:pokedexid
4
:pokemon_name
"Charmander"
:type1
"fire"
:type2 nil}
{:pokedexid
5
:pokemon_name
"Charmeleon"
:type1
"fire"
:type2 nil}
{:pokedexid
6
:pokemon_name
"Charizard"
:type1
"fire"
:type2 nil}])
(j
/
query db [
"SELECT * FROM pokemon"
])
(j
/
query db [
"SELECT * FROM pokemon"
] {:row
-
fn :pokemon_name})
(j
/
delete! db :pokemon [
"pokemon_name = ?"
"Charizard"
])
(j
/
query db [
"SELECT * FROM pokemon"
] {:row
-
fn :pokemon_name})
We hope this tutorial helped you to get started with using Progress DataDirect JDBC drivers with Clojure. Feel free to contact us in case of any questions or issues, we will be happy to help.